JavaScript - Shift Key & Any Key Input Event
Introducing a simple code example in JavaScript that implements an event triggered when the Shift key is pressed along with any other key.
In HTML, a <div> tag with the ID 'target' is designated as a target for detecting key inputs.
The code example enables focus by specifying 'tabindex', allowing the execution of key input events.
This is unnecessary when targeting text input forms or the entire 'document'.
<div id="target" tabindex="0">Press Shift and any other key</div>
In CSS, the size for the event target is set.
#target {
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
padding: 10px;
color: white;
width: 250px;
height: 250px;
border-radius: 50%;
text-align: center;
cursor: pointer;
background-color: #4b0082;
}
/* Specify the outline when focused */
#target:focus {
outline: 2px solid #000fff;
}
The target element is specified using querySelector()
, and 'addEventListener()
' is used to set up the 'keydown' event, triggered when a key is pressed.
The code uses conditions such as 'event.key != 'Shift'' to check if the pressed key is not the Shift key and 'event.shiftKey' to verify if the Shift key is already pressed to execute the specified action.
Similarly, 'keyup' is used to clear the displayed content when any key is released.
// Output area
let target = document.querySelector('#target');
target.addEventListener('keydown', () => {
// Prevent default action
event.preventDefault();
// Check if Shift key is pressed
if (event.key != 'Shift' && event.shiftKey) {
// Within the target element
target.innerHTML = 'Shift + ' + event.key;
}
});
target.addEventListener('keyup', () => {
// Clear the target display
target.innerHTML = 'Press Shift and any other key';
});
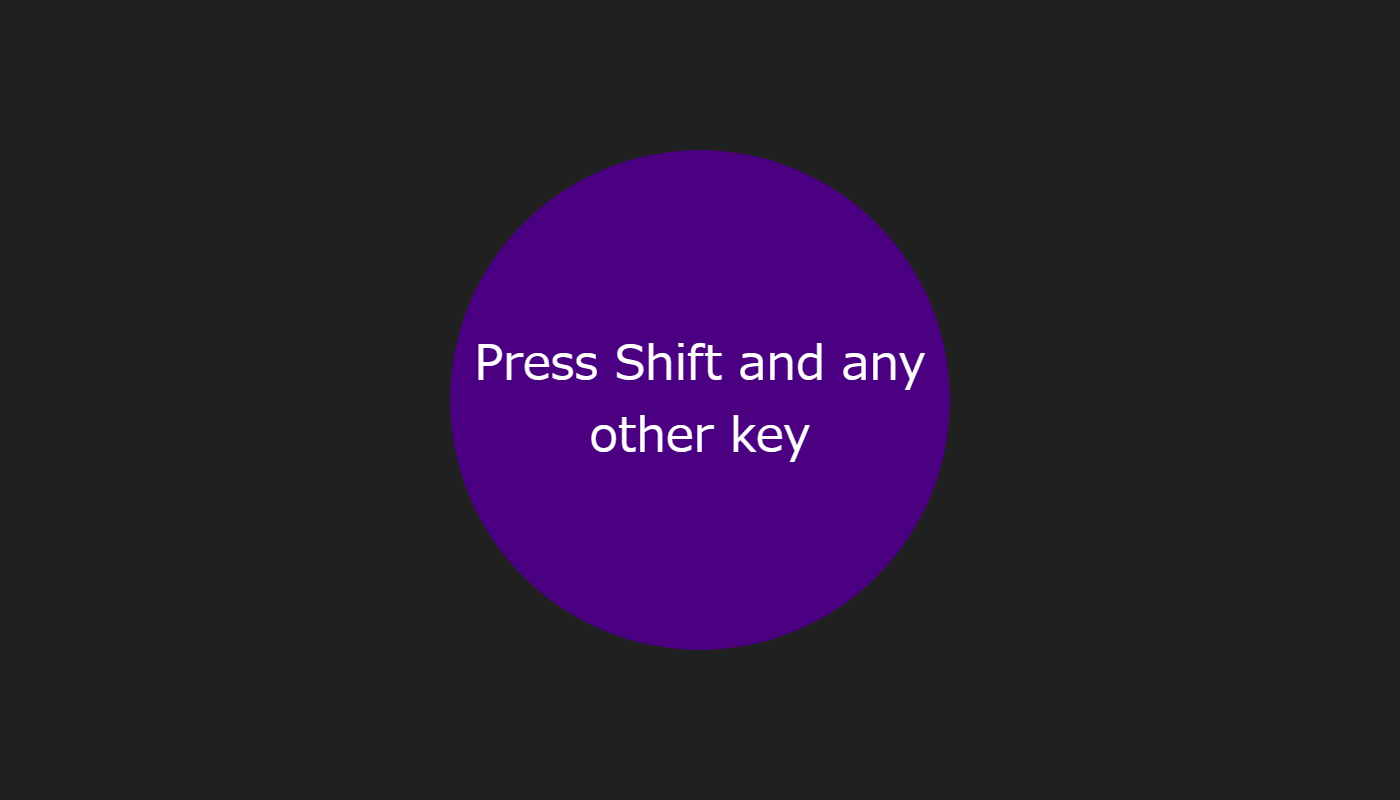