JavaScript - Drag and Drop Event
A simple code example to implement drag and drop events for elements using JavaScript.
In the HTML, a <div> tag is specified with the ID 'target' to detect drag and drop events. Additionally, multiple elements with the class 'drop-area' are set as the drop zones.
<div class="drop-area">
<div id="target" class="circle" draggable="true"></div>
</div>
<div class="drop-area"></div>
<div class="drop-area"></div>
In CSS, the sizes for the target and drop areas are defined to handle drag and drop events for elements.
.area, .drop-area {
display: flex;
justify-content: center;
align-items: center;
width: 120px;
height: 120px;
border: solid 1px #FFF;
margin: 10px;
}
.circle {
width: 100px;
height: 100px;
background: #ff69b4;
border-radius: 50%;
cursor: pointer;
}
Using 'addEventListener()
' with 'dragstart' to implement the drag start event for elements. During the drag start event, the dragged element is set using 'event.dataTransfer'.
For the 'dragover' event, it's necessary to prevent the default behavior to execute the drop action.
In the 'drop' event, the 'event.dataTransfer.getData('text')' retrieves the 'id' set to the element being dragged, and 'event.target.appendChild()
' moves the element to the drop area.
// Drop areas
let dropArea = document.querySelectorAll('.drop-area');
// Drag start
document.querySelector('#target').addEventListener('dragstart', () => {
// Set the dragged element
event.dataTransfer.setData('text', event.target.id);
});
// Drop
dropArea.forEach(element => {
element.addEventListener('dragover', () => {
// Prevent the default behavior
event.preventDefault();
});
element.addEventListener('drop', () => {
// Prevent the default behavior
event.preventDefault();
// Get the dragged element
const dropItem = document.querySelector('#' + event.dataTransfer.getData('text'));
// Move the dragged element
event.target.appendChild(dropItem);
});
});
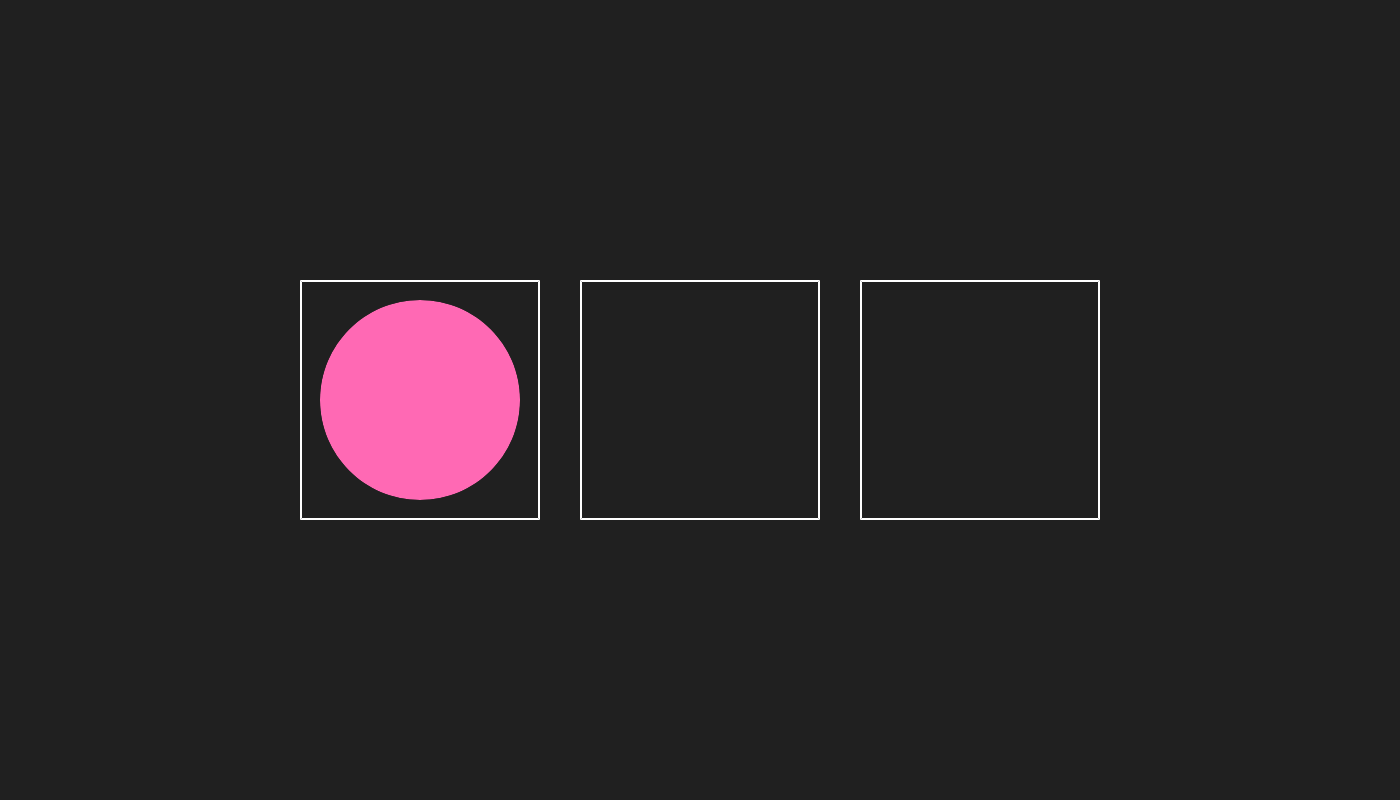