JavaScript - Key Press and Hold Event
A basic JavaScript code example for detecting when a specified key is pressed and held.
The HTML has a <div> tag with ID "target" that can detect key presses.
The "tabindex" attribute allows focus for detecting key events. Not needed for text inputs or document.
<div id="target" tabindex="0">Press and hold the spacebar</div>
The CSS sets the size for the event target element.
#target {
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
padding: 10px;
color: white;
width: 250px;
height: 250px;
border-radius: 50%;
text-align: center;
cursor: pointer;
user-select: none;
transition: 1s;
background: #dc143c;
}
The target element is selected with querySelector()
. The keydown event listener checks for the spacebar key code of 32.
A timer is set to trigger the hold event after a duration without the key being released.
The keyup listener clears the timer when released.
// Target Element
let target = document.querySelector('#target');
// Event timer ID
let eventTimerId;
// Key Down Event
target.addEventListener('keydown', () => {
// Check for spacebar
if (event.keyCode == 32) {
// Prevent default action
event.preventDefault();
// Set timer for hold duration
eventTimerId = setTimeout(function () {
// Code when hold event triggers
if (window.getComputedStyle(target).borderRadius !== '0%') {
target.style.borderRadius = '0%';
} else {
target.style.borderRadius = '50%';
}
}, 1000);
}
});
// Key Up Event
target.addEventListener('keyup', () => {
// Check for spacebar
if (event.keyCode == 32) {
// Clear event timer
clearTimeout(eventTimerId);
}
});
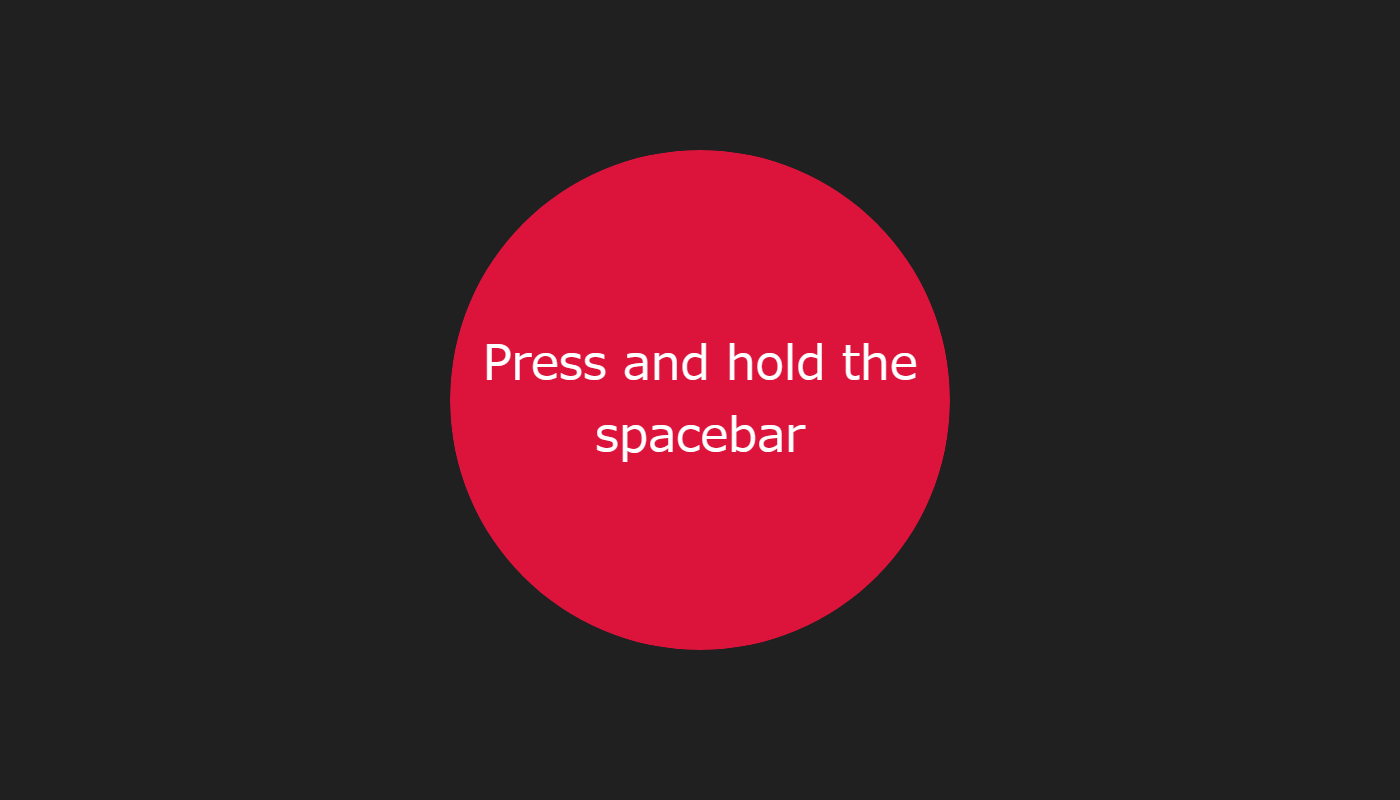