JavaScript - Long-Press Click Event
This presents a simple code example in JavaScript demonstrating the implementation of an event triggered by a long press of the left mouse button.
In HTML, a <div> tag with the ID 'target' is specified to detect long-press clicks.
<div id="target">Long-press<br>for 1 second</div>
The CSS sets the size for the event target.
#target {
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
padding: 10px;
color: white;
width: 250px;
height: 250px;
border-radius: 50%;
text-align: center;
cursor: pointer;
user-select: none;
transition: 1s;
background: #dc143c;
}
The target element is specified using querySelector()
. An 'mousedown' event is used via addEventListener()
to detect the pressing of the left-click button.
The shape is altered by referencing the 'border-radius' of the target element upon completion of the event timer.
// Target element
let target = document.querySelector('#target');
// Event timer ID
let eventTimerId;
// Mouse down event
target.addEventListener('mousedown', () => {
// Set the timer and create the eventTimerId
// Time considered for a long-press (in milliseconds)
eventTimerId = setTimeout(function () {
// Actions when the left mouse button is long-pressed
if (window.getComputedStyle(target).borderRadius !== '0%') {
target.style.borderRadius = '0%';
} else {
target.style.borderRadius = '50%';
}
}, 1000);
});
// When the left mouse button is released
target.addEventListener('mouseup', () => {
// Clear the event timer
clearTimeout(eventTimerId);
});
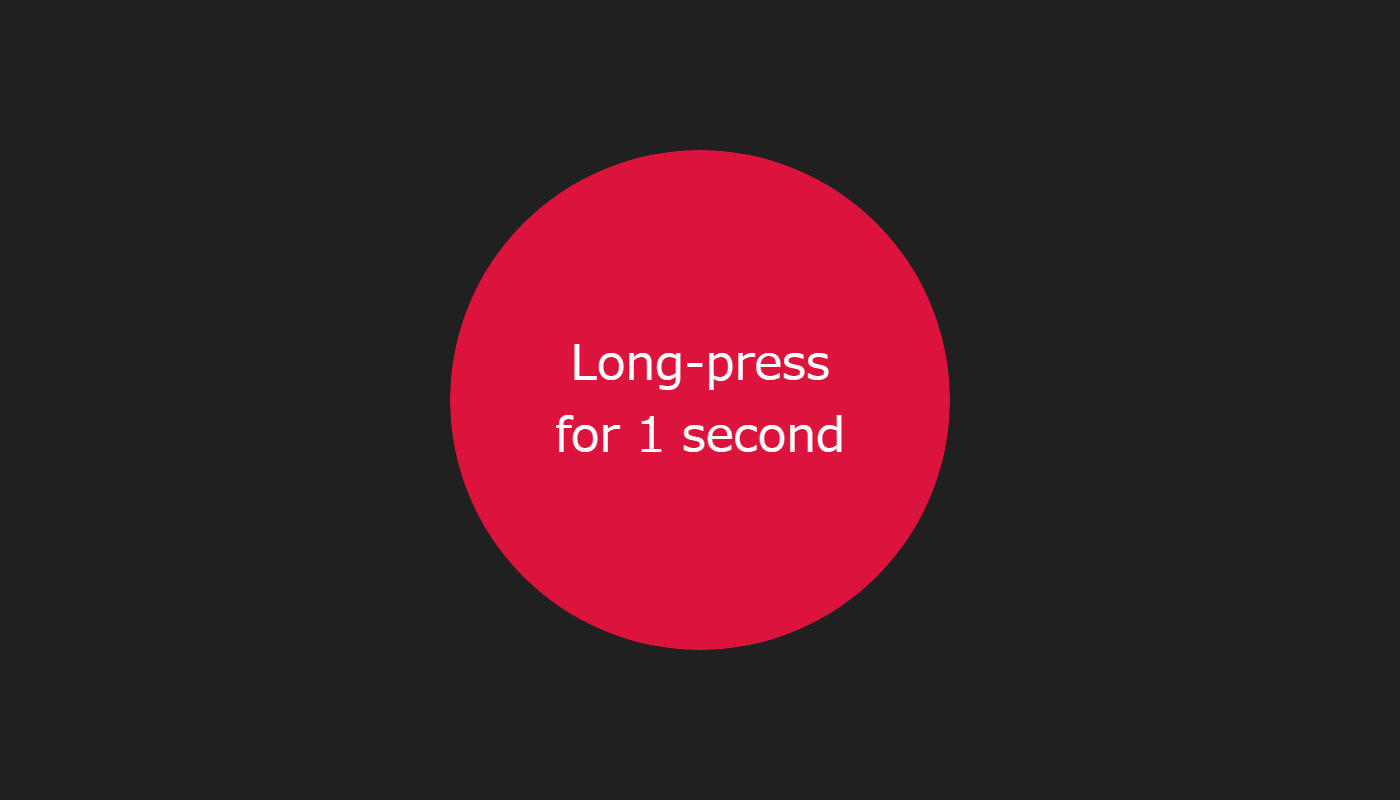