JavaScript - Focus switch event
Introducing a simple code example in JavaScript that implements events triggered when focusing on or removing focus from form elements.
In the HTML, an <input type="text"> element with the ID 'target' is used to detect focus changes.
When the target is not a form element, specifying the HTML attribute 'tabindex' allows focusing.
<div>
<p id="output">Not focused.</p>
<input type="text" id="target">
</div>
In CSS, the size for the event target is set.
Additionally, CSS allows specifying styles for the 'focus' pseudo-class when the element is clicked to focus.
#target {
width: 250px;
margin: 5px;
padding: 10px;
font-size: 24px;
}
#output {
font-size: 20px;
}
/* Specify style when focused */
#target:focus {
/* outline: 2px solid #000fff; */
}
The element is specified using querySelector()
, and 'addEventListener()
' is used to detect the 'focus' event when the element gains focus.
Additionally, it detects the 'blur' event when the focus is removed.
This code example alters the text in the output area when each event is detected.
// Form element to target
let target = document.querySelector('#target');
// Output area
let output = document.querySelector('#output');
target.addEventListener('focus', () => {
// Actions when focused
output.innerHTML = 'Focused.';
});
target.addEventListener('blur', () => {
// Actions when focus is removed
output.innerHTML = 'Not focused.';
});
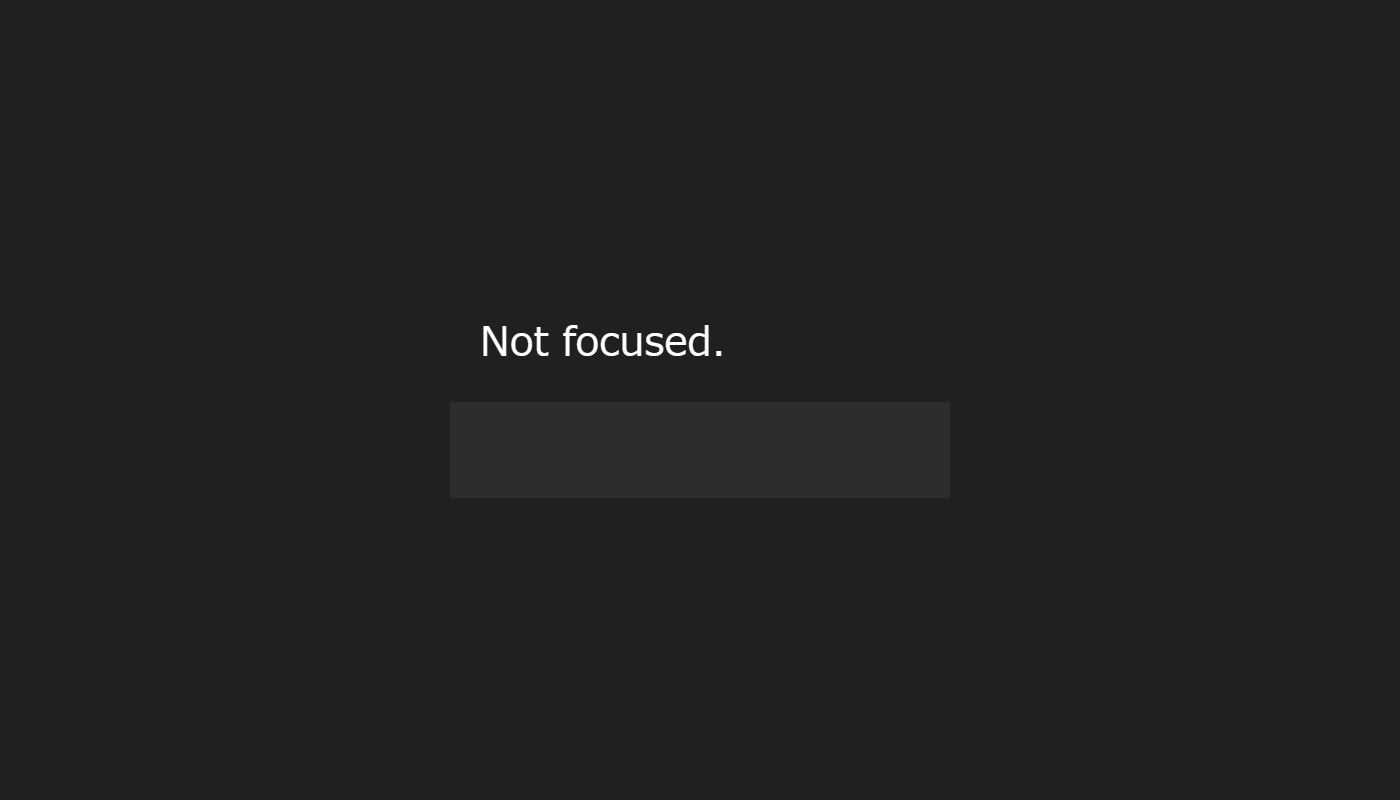