JavaScript - Detect start and end of CSS animation
A JavaScript code example for detecting the start and end of a CSS animation.
The HTML has a div with id "#target" to detect animation on.
<div class="item-container">
<div id="target"></div>
<p id="output">Waiting for animation</p>
</div>
The CSS has a simple rotation animation on the target element.
The animation starts after 3 seconds.
#target {
width: 100px;
height: 100px;
background: #4169e1;
animation: rotate-anim 3s 3s linear;
}
@keyframes rotate-anim {
0% {
transform: rotate(0deg);
}
100% {
transform: rotate(360deg);
}
}
.item-container {
width: 100%;
margin: 20px;
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
gap: 10px;
}
#output {
padding: 10px;
width: 100%;
text-align: center;
}
The animation start and end events are implemented on the target element using addEventListener()
.
"animationstart" and "animationend" can be specified to execute on each.
// Detect CSS animation start
document.querySelector('#target').addEventListener(`animationstart`, () => {
document.querySelector('#output').textContent = 'Animation started';
});
// Detect CSS animation end
document.querySelector('#target').addEventListener(`animationend`, () => {
document.querySelector('#output').textContent = 'Animation ended';
});
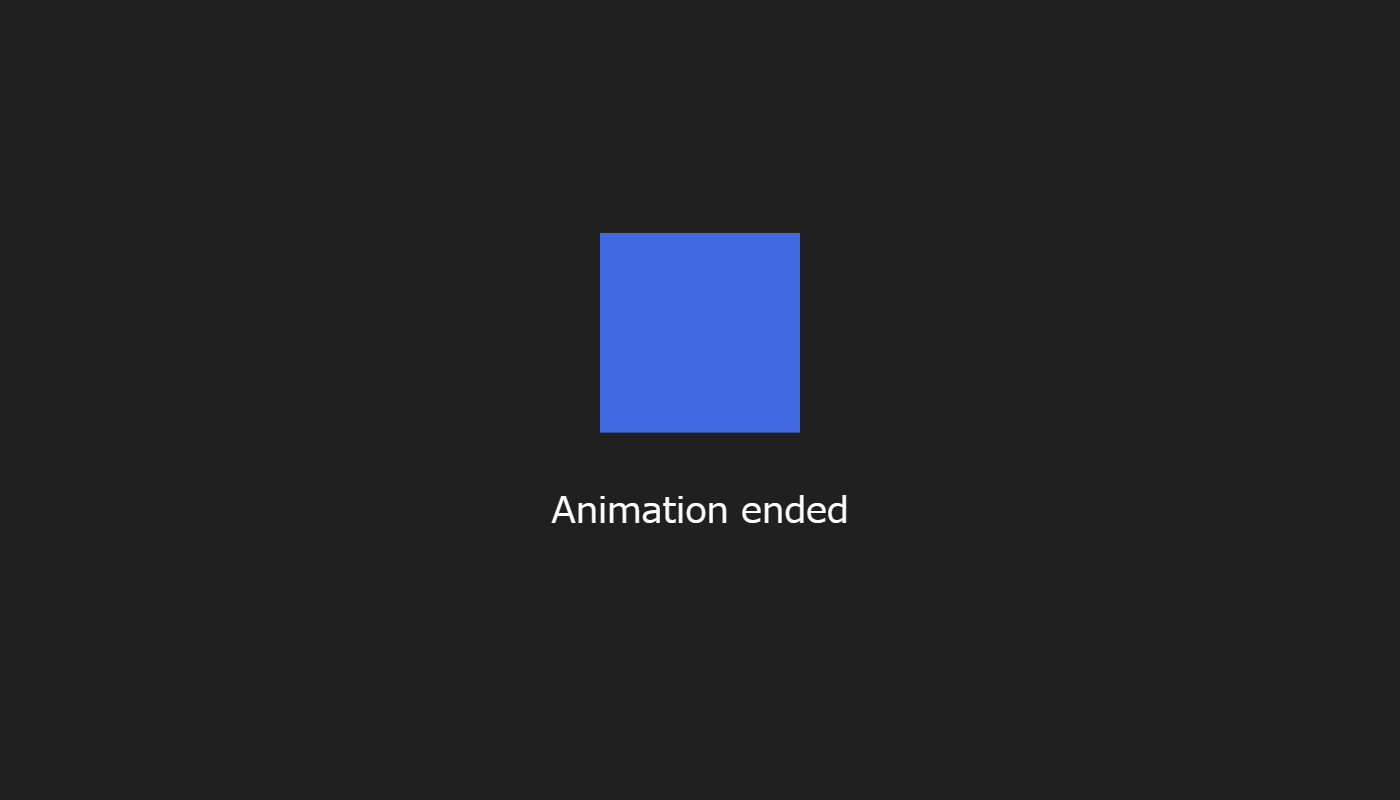
TitleJavaScript - Detect start and end of CSS animation
CategoryJavaScript
Created
Update
AuthorYousuke.U